CSCI 241 Labs: Lab 7
Static Methods with Triangles
Review these instructions
before
beginning the exercises.
There are 4 checkpoints
,
plus the
clean-up checkpoint, in this lab.
You and your partner should work together using just one of your
accounts.
If you need help with any exercise, raise your hand.
Getting Started
Copy the directory /home/student/Classes/Cs241/Labs/Lab07
to your account.
Change directories into Lab07
and start BlueJ by entering bluej&.
Online Documentation Review
As you have seen, Java programmers need to find details about the pre-built Java classes they use. We
have used classes that are both standard (included with the Java
language, such as String
and Scanner)
and non-standard (added for specific purposes,
such as the
ACM package of classes we have used for drawing circles in previous lab
exercises).
When you have an operating
system such as Microsoft Windows installed on a machine, the main
screen you see is called a 'desktop'. Using Gnome, you have the
potential to work with four 'virtual' desktops (in other words, there
are four desktops available, and you can see one at a time).
Gnome calls these workspaces.
An easy way to keep documentation handy is to have Firefox running in one
workspace while you run BlueJ in a different workspace.
In case you don't know how to do this:
- Examine the narrow panel in the lower-right corner of your screen.
You'll see a 1/4, which indicates you are in workspace #1
(out of a total of 4).
-
Click on each of the four workspaces in
turn. Each choice represents a different virtual desktop, and when you
click on one, your monitor screen shows the contents of that particular
desktop.
Return to the desktop in which
you are running BlueJ. Click on one of the three other workspaces
that are empty and start running Firefox.
In Firefox, go to your
course web page: www.cs.uwp.edu/Classes/Cs241.
In the Course
Related Documents and Websites
section, we have included links to documentation for both the standard
Java classes and also for the ACM library.
Click on "Java Platform Standard Edition & Java Development Kit
Version 11 API Specification". This document is the API
Application Programming Interface) specification for the version of
the Java language that we are using.
Find the java.base module in the table and click it.
The java.base module holds the most commonly used classes
including all that we will need this semester.
Find the java.lang
package in the table and click it.
A general summary of the java.lang package appears in the
introductory paragraphs. Scroll down to the Class Summary
section. You now see all the classes
contained in the java.lang
package. Examine the list; what looks familiar?
We'll be
asking you about which classes and methods you have used in the next
checkpoint.
Click the Integer
class
to see its details. Review its documentation. What
have you used from it?
Now, look at the details about the Math
class. What do you notice about it? How many
overloaded methods do you see?
1
Show us that you found this package and its classes, and answer
these questions:
- Which classes in java.lang
have you already used this semester? Which methods inside
those
classes?
- Remember how you learned to extract an int value from a String?
You wrote a line that looked like:
int x = Integer.parseInt(xString);
To calculate a square root, you write a line that looks like:
double y = Math.sqrt(number);
How are the formats of right-hand sides of these two lines similar to each other?
- Which methods in the Math
class are overloaded? How does Java tell them apart?
- There are three methods
that "round" numbers in the Math
class. They are: round(), ceil()
and floor().
How do they differ?
Triangle sides
Move back to the desktop containing BlueJ and open the
Lab07 project. In this project, open the Triangle
class in the editor window. This partially-written class contains
various calculations about a triangle, such as that seen in this
diagram:
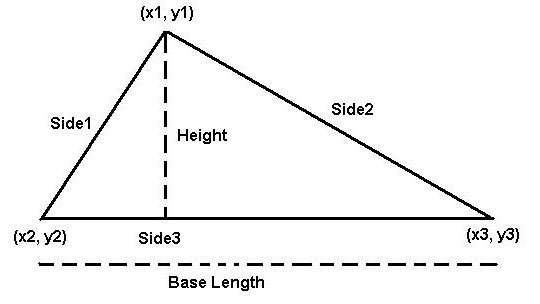
When the program runs completely, the main() method will ask the user
for three sets of (x,y) coordinates. It will then
be able to calculate the length of each side. Once you have the
lengths of the sides, all kinds of things can be calculated.
The first set of changes you need to make fall within the main() method. For this
checkpoint, complete the following:
- Comments in the code tell you which variables are missing in the
declarations. Complete those declarations, thinking carefully
about their data types. Initialize each variable to zero.
- Add code to calculate the height of the triangle. The
height of this program's triangle will be the absolute value of the
difference in y-coordinates between point 1 and point 2. Use the
appropriate Math class
method to help you with this calculation.
Not much more can be done until you calculate the length of each
side.
The formula for the distance between two points (x1, y1) and (x2,
y2) can be written as:
-

Here are 2 sample triangles and their points as would match up in the
diagram:
- Triangle A
- (x1,y1) = (0,2)
- (x2,y2) = (-1,0)
- (x3,y3) = (3,0)
- Triangle B
- (x1,y1) = (0,2)
- (x2,y2) = (0,0)
- (x3,y3) = (2,0)
Because this code will be used 3 times, it makes sense to ask a method
to do the calculation for us:
- Complete the method named calcSideLength(int, int, int, int),
which is already in the
code. It is written as a stub,
which means that the signature is complete but the body of the code
needs work. Use the formula above to calculate the value
to be returned to the caller, which is the main() method.
Which method in the Math
class helps you this time?
- Right-click on the class in BlueJ's project window to execute the
method with the points from triangles A and B, listed above.
For triangle A, you should get: side1 = 2.24, side2 = 3.61,
side3 = 4.00
For triangle B, you should get: side1 = 2.00, side2 = 2.83, side3 =
2.00
What would you expect to get for a side length if the x-values
are the same, and the y-values are different?
- Once you have tested this method by itself, uncomment
the lines in the main()
method that fill in the distances for each side. Does it run
correctly?
- Add lines to your main()
method to print all your newly calculated values. Don't forget to
include text next to each number so we know what it is.
2
Show us the code you have written and run it for us with triangles A
and B.
Lengths, Perimeter and Area
More calculations! Now that we have determined the sides of
the triangle, we can write other code for calculations that depend on
that information. Specifically, we want you to:
- Calculate the perimeter of the triangle by adding all of the side
lengths together. This code should be done inside the main() method.
- Set the base length of the triangle. It is defined as the
same as the length of side 3.
- Write a new static method named calcArea(double, double) which
takes
the base length and height as parameters, and returns the area.
The formula for the area of a triangle is:
- Test your calcArea()
method in BlueJ's project window by right-clicking on the Triangle
class. Use the points from triangles A and B above.
For triangle A, you should get: area = 4.00
For triangle B, you should get: area = 2.00
- Add a method call to your main()
method that will place the value this method returns into your area variable.
- Add your perimeter, base length and area to the part of your
program
that prints your triangle's information. Again, be sure to
include descriptive text next to each number.
3
Show us the code you have written and run it for us with triangles A
and B. What happens if you ask Java to run the calcArea()
method but you don't save the value it returns?
Boolean Methods
We can compare sides of triangle and use that information to classify
the triangle into one of these categories:
- An equilateral triangle
has all sides the same length.
- An isosceles
triangle has 2 sides of the same length.
- A scalene triangle has
sides of all different lengths.
We don't expect you to write all these methods for this checkpoint, but
you can do one of them.
- Write a method that will determine if the triangle is an
isosceles triangle. Its signature looks like:
isIsosceles(double, double, double)
Each of the parameters is a side length. The method should return
true if 2 of the sides are the
same
length, otherwise false.
As you know, comparing two double values
to each other using == or != is a bad idea.
Your method should return true
if
2 of the lengths are within 0.01 of each other. (Hint: find the differences, then
test the value of the difference in your condition.)
- Triangle B is not only a right triangle, it is also isosceles.
Triangle A is not. Try both sets of points when you test this method
directly.
- Once again, add code to invoke this method from main(). Use an if
-statement to print either "is isosceles" or "is not isosceles".
4
Show us the code you have written and run the program for us.
Would we be able to tell if the
triangle was equilateral by using this method, or would we need to
write a separate method to check all 3 sides?
Extra for Experts
Write methods to determine if the triangle is equilateral or scalene.
Hint: you can call any method from any other method; use
already-written
methods if that helps!
After the Lab
Return to Firefox, log onto Canvas and get your next
programming assignment.
Don't forget to log out from Canvas and close Firefox before you log
out.
5
Show us that you have logged out, cleaned up, turned off your monitor
and pushed in your chairs for this last checkpoint.
End of Lab